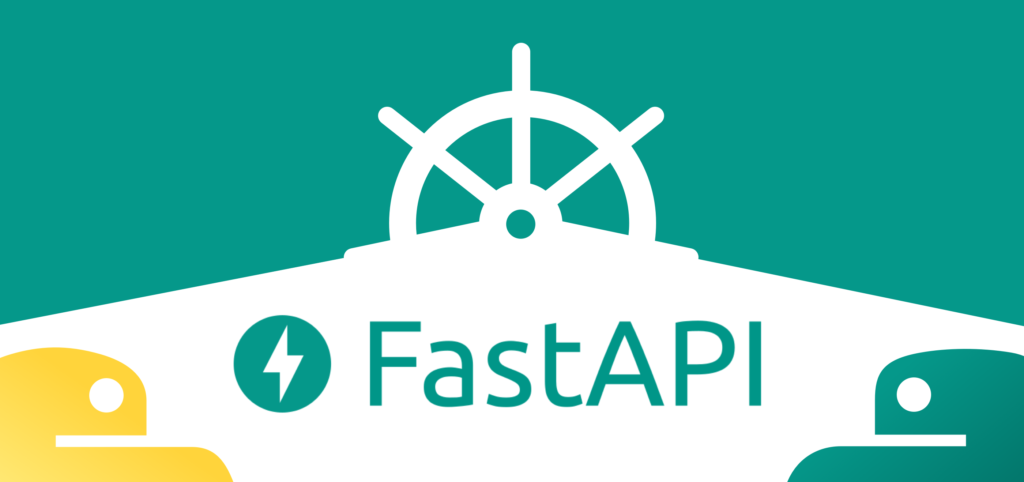
FastAPI is a modern, high-performance web framework for building APIs with Python. Here’s how you can get started quickly!
Step 1: Install FastAPI and Uvicorn
FastAPI is the web framework, and Uvicorn is the ASGI server used to run FastAPI applications.
Open your terminal or command prompt and run the following commands:
python -m pip install fastapi
pip install uvicorn
This will install FastAPI and Uvicorn on your system.
Step 2: Create Your First FastAPI Application
Create a Python file named myapi.py
and add the following code:
This code creates a FastAPI app with a single route (/
) that returns a JSON response with the message "Welcome to FastAPI!"
.
Step 3: Run Your FastAPI Application
Once your app is ready, you can run it using Uvicorn.
Run the following command in your terminal:
uvicorn myapi:app –reload
myapi:app
tells Uvicorn to look for theapp
object in themyapi.py
file.--reload
enables auto-reloading, so your server will automatically restart whenever you make changes to the code.
After running this command, Uvicorn will start the server, and your FastAPI application will be available at http://127.0.0.1:8000/
.
Step 4: Test Your API
Open your browser and go to http://127.0.0.1:8000/
. You should see the JSON response:
{
“name”: “First Data”
}
You can also explore FastAPI’s built-in interactive API documentation by visiting:
- Swagger UI:
http://127.0.0.1:8000/docs
- Redoc UI:
http://127.0.0.1:8000/redoc
Conclusion
That’s it! 🎉 You’ve successfully set up a basic FastAPI application. With just a few commands, you’re ready to start building APIs quickly and efficiently.
Stay tuned for more advanced topics in future posts!